To export a dataframe to Excel in Julia, you can use the XLSX.writetable()
function from the XLSX package. Here is an example:
Export DataFrame to Excel Example in Julia
using XLSX, DataFrames # define the dataframe df = DataFrame(A = [1, 2, 3], B = [4, 5, 6]) # write the dataframe to an Excel file XLSX.writetable("/Users/Myname/Documents/df1.xlsx", collect(DataFrames.eachcol(df)), DataFrames.names(df))
In this example, we first import the XLSX
and DataFrames
modules, which provide the functions we need to write a dataframe to an Excel file. Then, we define a dataframe with two columns, A
and B
.
Output
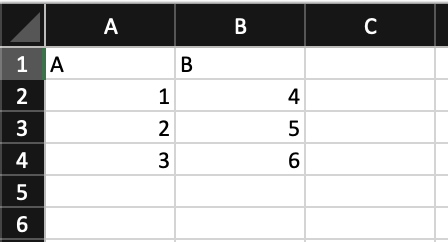
To write the dataframe to an Excel file, we use the XLSX.writetable()
function and provide the following arguments:
- The file path where the Excel file should be saved, e.g.
"/Users/Myname/Documents/df1.xlsx"
- The columns of the dataframe as an iterable collection, which we can obtain using the
DataFrames.eachcol()
function - The column names of the dataframe as an iterable collection, which we can obtain using the
DataFrames.names()
function
This will write the dataframe to the specified Excel file, using the column names as the first row and the column data as the subsequent rows. Note that this method does not allow you to specify any additional options, such as the sheet name or the range of cells to write to. If you want to customize the way the data is written to the Excel file, you can use the XLSX.writetable()
function directly and specify the options as keyword arguments. For more information and a complete list of options, see the documentation for the XLSX.writetable()
function.