In Python, the "ValueError: too many values to unpack (expected 2)" error occurs when you try to unpack more values from an iterable than what it has elements in it. Here's a simple example:
a, b = [1, 2, 3]
The above code will result in the following error:
ValueError: too many values to unpack (expected 2)
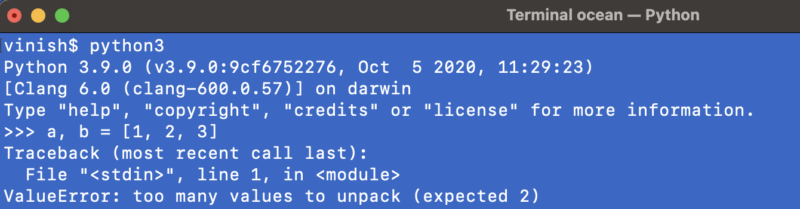
This error is telling you that there are 3 elements in the list [1, 2, 3]
but you are only trying to unpack 2 values a
and b
.
How to resolve the ValueError: too many values to unpack (expected 2)?
To resolve this error, you can either change the number of variables you are trying to unpack to match the number of elements in the iterable or you can change the number of elements in the iterable to match the number of variables you are trying to unpack.
Option 1: Change the number of variables
If you have more variables than the number of elements in the iterable, you can resolve the error by reducing the number of variables to match the number of elements.
a, b, c = [1, 2, 3]
Option 2: Change the number of elements in the iterable
If you have more elements in the iterable than the number of variables, you can resolve the error by reducing the number of elements in the iterable to match the number of variables.
a, b = [1, 2]
See also: ValuEerror: I/O operation on closed file in Python
Conclusion
The "ValueError: too many values to unpack (expected 2)" error occurs when you try to unpack more values from an iterable than what it has elements in it. To resolve this error, you can either change the number of variables you are trying to unpack to match the number of elements in the iterable or you can change the number of elements in the iterable to match the number of variables you are trying to unpack.
FAQs
This error occurs when you try to unpack more values from an iterable than what it has elements in it. For example, if you have a list with 3 elements and you try to unpack only 2 values from it, you will get this error.
To resolve this error, you can either change the number of variables you are trying to unpack to match the number of elements in the iterable or you can change the number of elements in the iterable to match the number of variables you are trying to unpack.
The "too many values to unpack" error occurs when you try to unpack more values from an iterable than what it has elements in it. On the other hand, the "not enough values to unpack" error occurs when you try to unpack more elements from an iterable than what it has values in it. For example, if you have a list with 2 elements and you try to unpack 3 variables from it, you will get the "not enough values to unpack" error.